Random walk: About
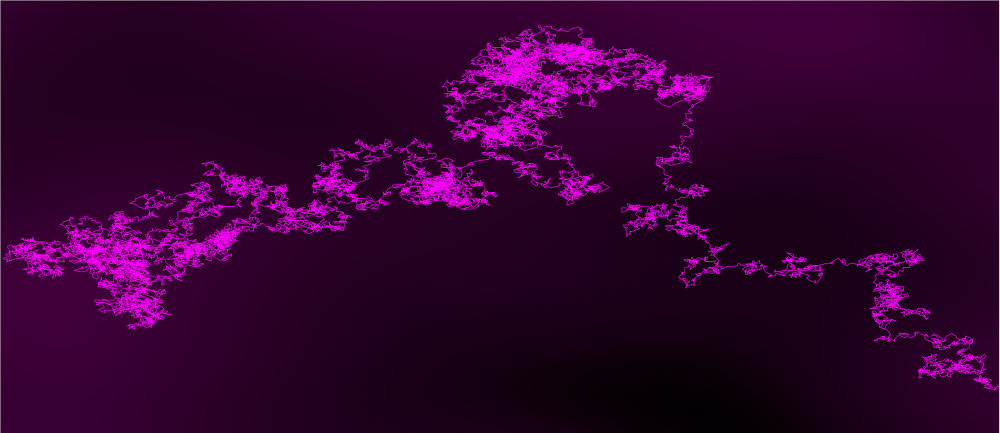
This is just the description bit. For reasons beyond comprehension, but something to do with child/parent HTML element sizes, the walk has to be viewed in a full browser window. So please visit and walk freely.
Just be aware that this is computationally intensive, and it may take a good few seconds to fully render. We generate a large object model within the graphing library we use. You may also be presented with a slow web page warning. Ignore it, or press “Wait”. It probably won’t work though if you’re browsing on a retro Apollo flight computer rebuild.
Our walk consists of 333,335 individual steps, in one of eight compass directions over a 2D plane (drunks do not just wander in lines at right angles). And we use the following right shift (>>
) trick to reduce our byte wise entropy consumption by 50%:-
// More bandwidth efficient technique for entropy consumption.
for (int i = OFFSET;
i < (OFFSET + NO_VALUES);
i++) {
int compass;
JsonArray newPoint;
compass = Byte.toUnsignedInt(entropy[i]) & 0b111; // 3 lsbits.
newPoint = createRoutePoint(compass);
route.add(newPoint);
compass = Byte.toUnsignedInt(entropy[i]) >> 5; // Other 3 msbits.
newPoint = createRoutePoint(compass);
route.add(newPoint);
}
We use an eight point compass like:-
// Returns a JSON array consisting of a single point.
private JsonArray createRoutePoint(int compass) {
switch (compass) {
case 0 ->
x += 1;
case 1 ->
x -= 1;
case 2 ->
y += 1;
case 3 ->
y -= 1;
case 4 -> {
x += 1;
y += 1;
}
case 5 -> {
x += 1;
y -= 1;
}
case 6 -> {
x -= 1;
y += 1;
}
case 7 -> {
x -= 1;
y -= 1;
}
}
JsonArray pointCoords = new JsonArray();
pointCoords.add(x);
pointCoords.add(y);
return pointCoords;
}
Yes, diagonal steps are $ \sqrt{2} $ times longer than those along the two axes. Blah. Remember that cryptography is art, not science. Also be aware that the Null Gamma Device is a raw entropy source. It is not a fully fledged TRNG, so it’s output entropy is biased. There is no randomness extractor. The bias is small and hard to notice from the random walks, but it’s there. So it’s kinda an entropy walk in reality, not a true random walk. Scientifically, it’s a biased random walk. But they’re still pretty with their fractal nature, and that’s what’s important.
And dwell on this: These walks are built from $\approx 1,000,000$ bits of truly random information, which is their approximate Kolmogorov complexity[1]. It’s a little less in reality as the entropy is biased, and thus ever so slightly compressible. If you kept looking at these images till the end of time, you’ll never see a repeat. Never, ever. Each one is absolutely unique. In terms of actual numbers, there are this many (approximately) possible wiggly variations of our walk:-
$$ \Large 10^{10^{5.479}} \text{ or } 9.9 \times 10^{301,029} $$
Or $ \approx 3.1 \times 10^{18,762} $ times the odds against a monkey typing Hamlet $(\approx 3.2×10^{282,303})$. Compare that to the $ \approx 10^{80} $ (100 quinvigintillion) atoms in the observable universe. Something that budding Entropists and Crypto-magés will feel very tingly about.

Notes:-
[1] It is mathematically impossible to accurately measure the Kolmogorov complexity of a set. So we hardly ever see what Kolmogorov complexity looks like. Until now. In the case of our walks, we do not need to measure their complexity a posteriori. We know their complexity because we construct them with a fixed amount of truly random (and hence very much incompressible) entropy. Therefore we know that approximately one million bits of entropy looks like this.